Scriptable Object Practice
Scriptable Objects are an extremely powerful tool that Unity provides developers. Described by Unity themselves, “a ScriptableObject is a data container that you can use to save large amounts of data, independent of class instances. One of the main use cases for ScriptableObjects is to reduce your Project’s memory usage by avoiding copies of values.” There are many possible use cases for these objects, and I’d like to quickly go over a recent use of them in my current top down shooter project.
One of the most important things for any game that has gun based combat is to have many different weapon choices, and while developing my top down shooter prototype project I needed to come up with a way to quickly create many weapon types. My solution, a scriptable object weapon and weapon attachment definition system!
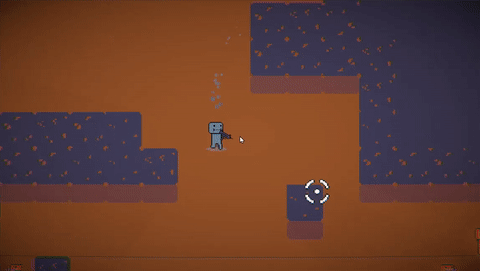
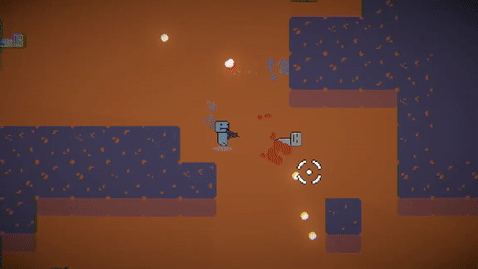
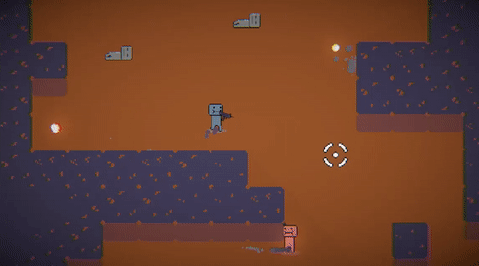
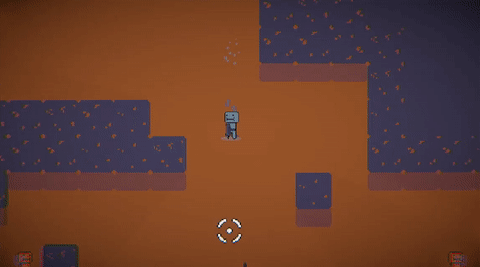
Architecture Overview
Main Architecture Components
Weapon Definition (ScriptableObject)
Weapon Attachments (ScriptableObject)
Weapon Interface (Monobehavior Script)
Weapon Prefab (Prefab / Prefab-Variant)
Weapon Definition
This is a ScriptableObject which defines the base behaviors of a weapon.
There are several properties which can be modified to define weapons ranging from simple pistols to rapid firing snake launchers.
Weapon Attachment
This is a ScriptableObject which defines several modifiers that can affect the behaviors of a weapon definition.
This can be used to create both Buffs, Upgrades, and DeBuffs.
Weapon Interface
This is a monobehavior script that processes how a weapon behaves based on several attached references.
Weapon Definition (ScriptableObject)
Weapon Attachments Array (ScriptableObject)
Projectile Starting Point (Gameobject Transform), this is a transform which allows you to position the origin point for where a projectile gets launched.
On successful firing of a projectile the Weapon Interface will also trigger an OnShoot Unity Event. This is used for auxiliary weapon behaviors such as:
Triggering the FMOD weapon audio event
Displaying weapon muzzle flash
Weapon Prefab
This is the shell that holds everything together. It contains two major components:
Sprite Renderer, used for storing a weapons visual appearance
Weapon Interface
Component reference chart
Constructing a default pistol for the prototype
While this system was developed for the purpose of creating player weapons, this system’s abstractness allows it to be used to even create weapons for the enemy ai.
Room for Improvement
This system is far from perfect and I think could definitely be further developed. Some ways that I can see this architecture being improved:
Not displaying variables that don’t pertain to a certain WeaponFireMode. ex. StaggeredFireRate doesn’t matter for a SEMIAUTO gun.
Allowing the weapon definition to hold and update the appearance of the weapon rather than needing to manually assign the view to the respective weapon sprite renderer.
Visualizing the WeaponBuffs on the weapon view
The WeaponInterface currently instantiates projectiles when it should be pulling them from a pool to improve performance.